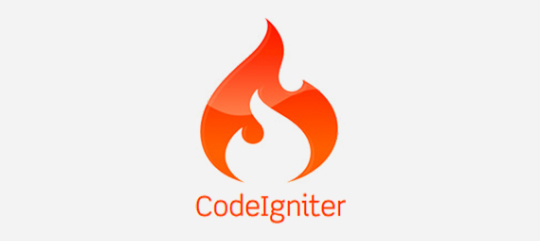
Google OAuth API is the simple and powerful way to integrate login system in the website. Google OAuth Login API let you allow the user to sign in the web application using their Google account without registration. The main advantage of Google login is the user can log in to the web application with their existing Google account without register account on the website. Nowadays almost all web users have a Google account, Sign-in with Google helps to increase the user’s subscription on your website.
Step1:-
Create Google API Console Project
-> Go to Google API Console.
-> Create project,Auth credentials for the project.
Step2:-
-> Download the latest version of codeigniter and extract into your root folder.
Step3:-
Create a google_config.php in /config folder, place below code.
defined('BASEPATH') OR exit('No direct script access allowed');
/* | To get API details you have to create a Google Project
| at Google API Console (https://console.developers.google.com)
| client_id string Your Google API Client ID.
| client_secret string Your Google API Client secret.
| redirect_uri string URL to redirect back to after login.
| application_name string Your Google application name.
| api_key string Developer key.
| scopes string Specify scopes */
$config['google']['client_id'] = 'Google_API_Client_ID';
$config['google']['client_secret'] = 'Google_API_Client_Secret';
$config['google']['redirect_uri'] = 'https://example.com/project_folder_name/user_authentication/';
$config['google']['application_name'] = 'Login to example.com';
$config['google']['api_key'] = '';
$config['google']['scopes'] = array();
Step4:-
Create Controller Auth.php In yor controllers/ folder.
if ( ! defined('BASEPATH')) exit('No direct script access allowed'); class Auth extends CI_Controller { function __construct() { parent::__construct(); $this->load->library('google');<br ?--> }
public function index(){
$data['google_login_url']=$this->google->get_login_url();
$this->load->view('home',$data);
}
public function oauth2callback(){
$google_data=$this->google->validate();
$session_data=array(
'name'=>$google_data['name'],
'email'=>$google_data['email'],
'source'=>'google',
'profile_pic'=>$google_data['profile_pic'],
'link'=>$google_data['link'],
'sess_logged_in'=>1
);
$this->session->set_userdata($session_data);
redirect(base_url());
}
public function logout(){
session_destroy();
unset($_SESSION['access_token']);
$session_data=array('sess_logged_in'=>0);
$this->session->set_userdata($session_data);
redirect(base_url());
}
}
Step5:-
Create A view home.php file in view forlder.
<html>
<head>
<title>Google authentication library for codeigniter</title>
<!-- Compiled and minified CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/0.96.1/css/materialize.min.css">
<link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/font-awesome/4.3.0/css/font-awesome.min.css">
<!-- Compiled and minified JavaScript -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.4/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/0.96.1/js/materialize.min.js"></script>
</head>
<body>
<div align="center">
<h2>Google authentication library for Codeigniter</h2>
</div>
<div class="container">
<div class="row">
<div class="col s12 m6 offset-m3 l6 offset-l3">
<?php
if($this->session->userdata('sess_logged_in')==0){?>
<a href="<?=$google_login_url?>"class="waves-effect waves-light btn red"><i class="fa fa-google left"></i>Google login</a>
<?php }else{?>
<a href="<?=base_url()?>auth/logout" class="waves-effect waves-light btn red"><i class="fa fa-google left"></i>Google logout</a>
<?php }
?>
</div>
</div>
<div class="row">
<?php if(isset($_SESSION['name'])){?>
<div class="col s12 m6 l4 offset-l3 " >
<div class="card ">
<div class="card-image waves-effect waves-block waves-light">
<img class="activator" src="<?=$_SESSION['profile_pic']?>">
</div>
<div class="card-content">
<span class="card-title activator grey-text text-darken-4"> <i class="material-icons"><?=$_SESSION['name']?></i></span>
</div>
<div class="card-reveal">
<span class="card-title grey-text text-darken-4"><?=$_SESSION['name']?><i class="material-icons right">close</i></span>
<p>Email Id: <?=$_SESSION['email']?></p>
</div>
</div>
</div>
<?php }?>
</div>
</div>
</body>
</html>
————————————————————-
Google Old Article
Google login in php.It is very easy at the same time,if somebody needs Google login in codeigniter websites.Just Follow the Steps you can easily login with Google in codeigniter.
Step 1:
Download the api Oauth2 Service package.
Note: If you are unable to find the package anywhere,please mail to me, I will send it to your mail id.
Step 2:
Download codeigniter latest version.
Step 3:
Extract and put the you are root folder.
Step 4:
Copy “src” folder from “apiOauth2Service” package and paste into codeigniter third_party folder.
Step 5:
Create config file into config folder name ‘google.php’.
Just write the below code
$config['google']['application_name'] = '';
$config['google']['client_id'] = '’;
$config['google']['client_secret'] = '’;
$config['google']['redirect_uri'] = 'http://www.domain.com/google/googleLoginSubmit';
$config['google']['api_key'] =’’;
Step 6:
Here go to Google api console and create an app and copy the client_id,client_secret,redirect_uri,api_key
Paste it into config file.Completed the main configuration part in codeigniter
Step 7:
Create one controller name google.php
Just write down the below code
<?php if ( ! defined('BASEPATH')) exit('No direct script access allowed');
class Google extends CI_Controller {
public function __construct() {
parent::__construct();
$this->config->load('google');
require APPPATH .'third_party/src/apiClient.php';
require APPPATH .'third_party/src/contrib/apiOauth2Service.php';
$cache_path = $this->config->item('cache_path');
$GLOBALS['apiConfig']['ioFileCache_directory'] = ($cache_path == '') ? APPPATH .'cache/' : $cache_path;
$this->client = new apiClient();
$this->client->setApplicationName($this->config->item('application_name', 'google'));
$this->client->setClientId($this->config->item('client_id', 'google'));
$this->client->setClientSecret($this->config->item('client_secret', 'google'));
$this->client->setRedirectUri($this->config->item('redirect_uri', 'google'));
$this->client->setDeveloperKey($this->config->item('api_key', 'google'));
$this->oauth2 = new apiOauth2Service($this->client);
}
/**
* Welcome index
*
* @access public
*/
public function index()
{
$data['auth'] = $this->client->createAuthUrl();
$this->load->view('google', $data);
}
public function googleLoginSubmit' (){
$this->input->get('code');
$this->client->authenticate();
$data1=$this->client->getAccessToken();
$data['user'] = $this->oauth2->userinfo->get();
echo "<pre>"; print_r($data);
//$this->load->view('google', $data);
}
}
?>
Step 8:
Create file in views name as google.php
Just write the below code
<?php
echo "Login with google";
?>
<br />
<a href="<?php echo $auth;?>">Google Login</a>
That is it!!! You can now login with Google in Codeigniter.
63 Comments
vandana
September 8, 2014A PHP Error was encountered
Severity: Notice
Message: Undefined property: google::$client
Filename: controllers/google.php
Line Number: 26
basanth
October 14, 2014can u send 0auth file to basanthboss@gmail.com
raghava
October 16, 2014Please send me api Oauth2 Service package.
If possible send me any sample code
admin
October 29, 2014Hi Raghava,
Here is the link for Oauth2 Sevice package. You can download and try as mentioned in the article. Feel free to contact if you have any issues.
https://github.com/thephpleague/oauth2-client
Thank you.
John Smith
October 27, 2014Hi Sudhakar
Please send me Oauth2 Service package. I have tried many other libraries, but any of them did not worked for me.
Thanks
John Smith
admin
October 29, 2014Hi,
Here is the link for Oauth2 Sevice package. You can download and try as mentioned in the article. Feel free to contact if you have any issues.
https://github.com/thephpleague/oauth2-client
Thank you.
Suvra Naha
October 29, 2014This code is very useful.But i don’t have the Oauth2 Service package.can you please send it.Thanks in advance..
admin
October 29, 2014Hi Suvra Naha,
Here is the link for Oauth2 Sevice package. You can download and try as mentioned in the article. Feel free to contact if you have any issues.
https://github.com/thephpleague/oauth2-client
Thank you.
chintan
October 30, 2014i don’t have the Oauth2 Service package.can you please send it.
siva
November 7, 2014Hi,
i am using Google login in codeigniter website.The App keeps asking for permission to “Have offline access” how to solve this problem…
Yogesh
November 7, 2014hi ,
please send me package, i did not find the package.
thanks
prasad
November 16, 2014Hi, Iam unable to create the app i.e., unable to create the Id,secret key and api key in the Google api. Please could you tell me how?
prasad
November 19, 2014HI, I have followed the above procedure but finally I am getting the error as:
Fatal error: require(): Failed opening required ‘application/third_party/src/apiClient.php’ (include_path=’.;C:\xampp\php\PEAR’) in C:\xampp\htdocs\project1\application\controllers\Google.php on line 6
prasad
November 19, 2014Hi, there is no apiClient.php file in src folder..!Please tell me where the “apiClient.php” file located in the ‘src’ folder?
abhishek
November 19, 2014Hi,
I have downloaded the library but src folder doesn’t contain apiClient.php and apiOauth2Service.php,which is causing error
Message: require(application/third_party/src/apiClient.php): failed to open stream: No such file or directory
. Am I missing something ?
Please help.
Thanks
sonu
November 26, 2014i need src folder of the api Oauth2 Service package.
ravi
November 28, 2014A PHP Error was encountered
Severity: Warning
Message: require(application/third_party/src/apiClient.php): failed to open stream: No such file or directory
Filename: controllers/google.php
Line Number: 6
A PHP Error was encountered
i’m getting this error
Severity: Warning
Message: require(application/third_party/src/apiClient.php): failed to open stream: No such file or directory
Filename: controllers/google.php
Line Number: 6
Elcio
December 1, 2014Please, send me the Oauth2service plugin
Thirumalai
December 15, 2014Send me the source folder that to be copied in the third_party folder
eswar
January 6, 2015Hi,
I have downloaded the library but src folder doesn’t contain apiClient.php and apiOauth2Service.php,which is causing error
Message: require(application/third_party/src/apiClient.php): failed to open stream: No such file or directory..
Dear Shilpa can uy plz mail that library…
kiran
January 13, 2015i need src folder pls mail me….
pravinkumar
February 3, 2015i need src folder of the api Oauth2 Service package.
Sham
February 9, 2015i need src folder of the api Oauth2 Service package.
rohini
February 10, 2015can you send oauth php client service package
Naga
February 23, 2015Please madam i need src folder of the api Oauth2 Service package.
nagesh
February 26, 2015Hi I am getting
A PHP Error was encountered
Severity: Warning
Message: include_once(application/libraries/google-api-php-client-master/src/Google/Client.php): failed to open stream: No such file or directory
Filename: controllers/user_authentication.php
Line Number: 15
this error.
can you please send me the oauth2 service package.
I am unable to find it.
My email is
nageshkatke@outlook.com
Thank You
sunil
April 30, 2015Hi,
Please send the package to sunilnagpal30@gmail.com
Thanks
Zypher
May 25, 2015Hi, the github link you provided lacks apiClient.php alot of people are looking for it, I hope you could help us!
ashitha
July 13, 2015Download the api Oauth2 Service package.
ashok sharma
July 15, 2015please provide me api Oauth2 Service package for login with google code
nandar
July 16, 2015Please send me api and now I am testing in my localhost/mmpropertylisting
nandar
July 16, 2015Please send me api and now I am testing on my localhost.
Amol
July 30, 2015please send me the package on amolj3064@gmail.com
Nagesh
August 6, 2015Hi,
Send me this script ,so i can implement in my website
manish katiyar
September 7, 2015Please sent me “api Oauth2 Service package”.
if You have.
Thank You
santanuadak
September 7, 2015please send
santanuadak
September 7, 2015santanuadak0@gmail.com
dhirendra
September 10, 2015hi
please send me the src folder to dksinghdhiru12@gmail.com
thanks
Balaji Dhumal
September 12, 2015hi..
please send me api Oauth2 Service package.
Srinivas
October 13, 2015I want Oauth2 API code, please send me ASAP.
aman
October 22, 2015Dont use this its not working anymore
Anil
October 27, 2015Please can you email me the Oauth2 Service package.
Anil
October 27, 2015Hi,
Thank you for this.
Please can you email me the package.
Thank you.
Anil
Vukomir
November 26, 2015Hi,
please send me the src folder
vukomir@os-ux.com
Thanks.
abdul
December 28, 2015I tried whole day, but i unable to get the correct src folder and files. kindly mail me the src folder
testaccnt0435@gmail.com
chandani
January 7, 2016hi,
please send Oauth2 Service package.
chandani.potenza@gmail.com
Thanks
Dharmendra Patil
January 12, 2016Hi I am getting
A PHP Error was encountered
Severity: Warning
Message: require(C:\wamp\www\ci_oauth2\application\third_party/src/apiClient.php): failed to open stream: No such file or directory
Filename: controllers/google.php
Line Number: 13
Backtrace:
File: C:\wamp\www\ci_oauth2\application\controllers\google.php
Line: 13
Function: _error_handler
File: C:\wamp\www\ci_oauth2\application\controllers\google.php
Line: 13
Function: __construct
File: C:\wamp\www\ci_oauth2\index.php
Line: 292
Function: require_once
My email is
dharmendrarpatil@gmail.com
Thank You
rohit
January 18, 2016pls send me the Oauth2 Service package.
dipali
January 27, 2016plz send api Oauth2 Service package
dipali
January 27, 2016Please madam i need src folder of the api Oauth2 Service package.
dipali
January 29, 2016Hiii
400. That’s an error.
Error: invalid_request
Missing required parameter: redirect_uri
Learn more
Request Details
That’s all we know.
This is problem does not solved plz help me
Arun Singh
March 16, 2016HI,
I am using using your Script , but there is error occured as
require(apps/third_party/src/apiClient.php): failed to open stream: No such file or directory
the file apiClient.php not found in src folder So can you send me it immeiatly.
Thanks In adwance.
Jerin
March 24, 2016I need oauth files
Sunil
September 14, 2016Hi,
Please send the package to
sunilkate111@gmail.com
Thanks
horse rider underwear
October 9, 2016As I site possessor I believe the content matter
here is rattling great , appreciate it for your hard work.
You should keep it up forever! Best of luck.
Mukesh
January 2, 2017Hi,
Please send the package to
mukeshdubey118@gmail.com
Thanks
puneet
July 23, 2017There is no apiClient.php in scr folder
mohit
August 31, 2017Hi,
Please send the package to
mohit2773@gmail.com
Thanks
akash shrimali
September 13, 2017please send apiOath2 folder
akash shrimali
September 13, 2017Hi,
Please send package to
akashshrimali1998@gmail.com
sneha
September 18, 2017can u send me the auth file to snehavijayan90@gmail.com
nirwan
August 13, 2018can you send me the package to nirwan.vi@gmail.com
Thanks
santhosh
September 4, 2018Fatal error: Class ‘src\GoogleApi\Service\ServiceResource’ not found in C:\xampp\htdocs\googleplus\application\third_party\src\GoogleApi\Contrib\apiOauth2Service.php on line 33
A PHP Error was encountered
Severity: Error
Message: Class ‘src\GoogleApi\Service\ServiceResource’ not found
Filename: Contrib/apiOauth2Service.php
Line Number: 33
Backtrace:
please rectify my error